Image Manipulate
A collection of useful functions to manipulate and change images. The image must be an instance of a SimpleITK image and the functions are mostly wrappers for SimpleITK image filters. Check SimpleITK filters for available methods of filtering, registration, etc.
- fredtools.mapStructToImg(img, RSfileName, structName, binaryMask=False, areaFraction=0.5, CPUNo='auto', displayInfo=False)
Map structure to image and create a mask.
The function reads a structName structure from the RS dicom file and maps it to the frame of reference of img defined as a SimpleITK image object. The created mask is an image with the same frame of reference (origin. spacing, direction and size) as the img with values larger than 0 for voxels inside the contour and values 0 outside. In primary usage, the function produces floating masks, i.e., the value of each voxel describes its fractional occupancy by the structure. It is assumed that the image is 3D and has a unitary direction, which means that the axes describe X, Y and Z directions, respectively. The frame of reference of the img is not specified, in particular, the Z-spacing does not have to be the same as the structure Z-spacing.
- Parameters:
img (SimpleITK Image) – Object of a SimpleITK 3D image.
RSfileName (string) – Path String to dicom file with structures (RS file).
structName (string) – Name of the structure to be mapped.
binaryMask (bool, optional) – Determine binary mask production using areaFraction parameter. (def. False)
areaFraction (scalar, optional) – Fraction of pixel area occupancy to calculate binary mask. Used only if binaryMask==True. (def. 0.5)
CPUNo ({'auto', 'none'}, scalar or None, optional) – Define if the multiprocessing should be used and how many cores should be exploited. It can be None, and then no multiprocessing will be used, a string ‘auto’, then the number of cores will be determined by os.cpu_count(), or a scalar defining the number of CPU cores to be used. (def. ‘auto’)
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a SimpleITK image describing a floating or binary mask.
- Return type:
SimpleITK Image
See also
cropImgToMask
crop image to mask boundary.
getDVHMask
calculate DVH for the mask.
Notes
1. the functionality of
gatetools
package has been tested, but it turned out that it does not consider holes and detached structures. Therefore, a new approach has been implemented2. The mapping is done for each contour separately and based on the direction (CW or CCW) of the contour, it is treated as an inclusive (mask, CW) or exclusive (hole, CCW) contour. The mapping of each contour is done in 2D, meaning slice by slice. The resulting image has the voxel size and shape the same as the input img in X and Y directions. The voxel size in the Z direction is calculated based on the contour slice distances, taking into account gaps, holes and detached contours. The shape of the image in the Z direction is equal to the contour boundings in the Z direction, enlarged by 1 px (
sliceAddNo
parameter). Such image mask is then resampled to the frame of reference of the input img. In fact, the resampling is applied only to the Z direction, because the frame of reference of X and Y directions are the same as the input img.
- fredtools.floatingToBinaryMask(imgMask, threshold=0.5, thresholdEqual=False, displayInfo=False)
Convert floating mask to binary mask.
The function converts an image defined as an instance of a SimpleITK image object describing a floating mask to a binary mask image, based on a given threshold.
- Parameters:
imgMask (SimpleITK Image) – An object of a SimpleITK image describing a binary mask.
threshold (scalar, optional) – The threshold to calculate the binary mask. (def. 0.5)
thresholdEqual (bool, optional) – Determines if the threshold is larger or larger of equal. If the parameter is true, then the threshold must be larger than 0. (def. False)
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a SimpleITK image.
- Return type:
SimpleITK Image
See also
mapStructToImg
mapping a structure to an image to create a mask.
- fredtools.cropImgToMask(img, imgMask, displayInfo=False)
Crop image to mask boundary.
The function calculates the boundaries of the imgMask defined as an instance of a SimpleITK image object describing a binary or floating mask and crops the img defined as an instance of a SimpleITK image object to these boundaries. The boundaries mean here the most extreme positions of positive values (1 for binary mask and above 0 for floating mask) of the mask in each direction. The function exploits the SimpleITK.Crop routine.
- Parameters:
img (SimpleITK Image) – An object of a SimpleITK image.
imgMask (SimpleITK Image) – An object of a SimpleITK image describing a mask.
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a SimpleITK image.
- Return type:
SimpleITK Image
See also
mapStructToImg
mapping a structure to an image to create a mask.
- fredtools.setValueMask(img, imgMask, value, outside=True, displayInfo=False)
Set value inside/outside mask.
The function sets the values of the img defined as an instance of a SimpleITK object that are inside or outside a binary or floating mask described by the imgMask, defined as an instance of a SimpleITK object describing a mask. The inside of the mask is defined for voxels with 1 for the binary mask and above 0 for the floating mask. The function is a simple wrapper for the SimpleITK.Mask routine.
- Parameters:
img (SimpleITK Image) – An object of a SimpleITK image.
imgMask (SimpleITK Image) – An object of a SimpleITK image describing a mask.
value (scalar) – value to be set (the type will be mapped to the type of img).
outside (bool, optional) – Determine if the values should be set outside the mask (where mask values are equal to 0) or inside the mask (where mask values are above 0) (def. True meaning outside the mask)
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a SimpleITK image.
- Return type:
SimpleITK Image
See also
mapStructToImg
mapping a structure to an image to create a mask.
- fredtools.resampleImg(img, spacing, interpolation='linear', splineOrder=3, displayInfo=False)
Resample image to other voxel spacing.
The function resamples an image defined as an instance of a SimpleITK image object to different voxel spacing using a specified interpolation method. The assumption is that the ‘low extent’ is not changed, i.e. the coordinates of the corner of the first voxel are preserved. The size of the interpolated image is calculated to fit all the voxels’ centers in the original image extent. The function exploits the SimpleITK.Resample routine.
- Parameters:
img (SimpleITK Image) – An object of a SimpleITK image.
spacing (array_like) – New spacing in each direction. The length should be the same as the img dimension.
interpolation ({'linear', 'nearest', 'spline'}, optional) – Determine the interpolation method. (def. ‘linear’)
splineOrder (int, optional) – Order of spline interpolation. Must be in the range 0-5. (def. 3)
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a SimpleITK image.
- Return type:
SimpleITK Image
- fredtools.sumImg(imgs, displayInfo=False)
Sum list of images.
The function sums an iterable (list, tuple, etc.) of images defined as instances of a SimpleITK image object. The frame of references of all images must be the same.
- Parameters:
imgs (iterable) – An iterable (list, tuple, etc.) of SimpleITK image objects.
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a SimpleITK image.
- Return type:
SimpleITK Image
- fredtools.imgDivide(imgNum, imgDen, displayInfo=False)
Divide two images.
The function divides two images images defined as instances of a SimpleITK image object. The frame of references of both images must be the same. For a given voxel it returns:
NaN value if any of the numerator or denominator voxel value is NaN
0 value if denominator voxel value is zero
Quotient for all other cases
- Parameters:
imgNum (SimpleITK Image) – An object of a SimpleITK image describing numerator.
imgDen (SimpleITK Image) – An object of a SimpleITK image describing denominator.
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a SimpleITK image.
- Return type:
SimpleITK Image
- fredtools.sumVectorImg(img, displayInfo=False)
Sum vector image.
The function sums all elements of a vector in a vector image defined as instances of a SimpleITK vector image object. The resulting image has the same frame of reference but is a scalar image.
- Parameters:
img (SimpleITK Vector Image) – An object of a SimpleITK vector image.
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
Object of a SimpleITK image.
- Return type:
SimpleITK Image
- fredtools.createEllipseMask(img, point, radii, displayInfo=False)
Create an Ellipse mask in the image field of reference.
The function creates an ellipse mask, defined with the center and radii in the frame of references of an image defined as a SimpleITK image object. Any dimension, i.e. 2D-4D, of the image is supported.
- Parameters:
img (SimpleITK Image) – An object of a SimpleITK image.
point (array_like) – A point describing the position of the center of the ellipse. The dimension must match the image dimension.
radii (scalar or array_like) – Radii of the ellipse for each dimension. It might be a scalar, then the same radii will be used in each direction.
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An instance of a SimpleITK image object describing a binary mask (i.e. type ‘uint8’ with 0/1 values).
- Return type:
SimpleITK Image
See also
mapStructToImg
mapping a structure to an image to create a mask.
setValueMask
setting values of the image inside/outside a mask.
cropImgToMask
crop an image to mask.
createCylinderMask
create a cylinder mask.
createConeMask
create a cone mask.
- fredtools.createConeMask(img, startPoint, endPoint, startRadius, endRadius, displayInfo=False)
Create a cone mask in the image field of reference.
The function creates a cone mask, defined with starting and ending points and radii in the frame of references of an image defined as a SimpleITK image object describing a 3D image. Only 3D images are supported.
- Parameters:
img (SimpleITK Image) – Object of a SimpleITK 3D image.
startPoint (array_like) – 3-element point describing the position of the center of the first cone base.
endPoint (array_like) – 3-element point describing the position of the center of the second cone base.
startRadius (scalar) – Radious of the first cone base.
endRadius (scalar) – Radious of the second cone base.
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An instance of a SimpleITK image object describing a binary mask (i.e. type ‘uint8’ with 0/1 values).
- Return type:
SimpleITK Image
See also
mapStructToImg
mapping a structure to an image to create a mask.
setValueMask
setting values of the image inside/outside a mask.
cropImgToMask
crop an image to mask.
createCylinderMask
create a cylinder mask.
createEllipseMask
create an ellipse mask.
- fredtools.createCylinderMask(img, startPoint, endPoint, radious, displayInfo=False)
Create a cylindrical Mask in the image field of reference
The function creates a cylindrical mask with a given radious and height calculated from the starting and ending points of the cylinder in the frame of references of an image defined as a SimpleITK image object describing a 3D image. Only 3D images are supported. For instance, the routine might help make a geometrical acceptance correction of a chamber used for Bragg peak measurements. The routine was adapted from a GitHub repository: https://github.com/heydude1337/SimplePhantomToolkit/.
- Parameters:
img (SimpleITK Image) – Object of a SimpleITK 3D image.
startPoint (array_like) – 3-element point describing the position of the center of the first cylinder base.
endPoint (array_like) – 3-element point describing the position of the center of the second cylinder base.
radious (scalar) – Radious of the cylinder.
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An instance of a SimpleITK image object describing a mask (i.e. type ‘uint8’ with 0/1 values).
- Return type:
SimpleITK Image
See also
mapStructToImg
mapping a structure to an image to create a mask.
setValueMask
setting values of the image inside/outside a mask.
cropImgToMask
crop an image to mask.
createConeMask
create a cone mask.
createEllipseMask
create an ellipse mask.
- fredtools.getImgBEV(img, isocentrePosition, gantryAngle, couchAngle, defaultPixelValue='auto', interpolation='linear', splineOrder=3, displayInfo=False)
Transform an image to Beam’s Eye View (BEV).
The function transforms a 3D image defined as a SimpleITK 3D image object to the Beam’s Eye View (BEV) based on the given isocentre position, gantry angle and couch rotation, using a defined interpolation method. The BEV Field of Reference (FOR) means that the Z+ direction is along the field (along the beam of relative position [0,0]) and X/Y positions are consistent with the DICOM and FRED Monte Carlo definitions.
- Parameters:
img (SimpleITK 3D Image) – Object of a SimpleITK 3D image.
isocentrePosition (array_like, (3x1)) – Position of the isocentre with respect to the img FOR.
gantryAngle (scalar) – Rotation of the gantry around the isocentre position in [deg].
couchAngle (scalar) – Rotation of the couch around the isocentre position in [deg].
defaultPixelValue ('auto' or scalar, optional) – The value to fill the voxels with, outside the original img. If ‘auto’, then the value will be calculated automatically as the minimum value of the img. (def. ‘auto’)
interpolation ({'linear', 'nearest', 'spline'}, optional) – Determine the interpolation method. (def. ‘linear’)
splineOrder (int, optional) – Order of spline interpolation. Must be in the range 0-5. (def. 3)
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a transformed SimpleITK 3D image.
- Return type:
SimpleITK 3D Image
Notes
The basic workflow follows:
translate the image to the isocentre as to have the isocentre at zero position,
rotate the couch around the isocentre,
rotate the gantry around the isocentre,
rotate and flip the image to get BEV.
Note that the isocentre of the transformed image is at the zero point.
Note that the isocentre defined in the delivery sequence of the FRED rtplan is a negative isocentre defined in the DICOM RN plan, the couch rotation defined in the delivery sequence of the FRED rtplan is a negative couch rotation defined in the DICOM RN plan, but the gantry rotation defined in the delivery sequence of the FRED rtplan is equal to the gantry rotation of the DICOM RN plan.
- fredtools.setIdentityDirection(img, displayInfo=False)
Set an identity direction for the image.
The function sets an identity direction of an image defined as an instance of a SimpleITK image.
- Parameters:
img (SimpleITK Image) – An object of a SimpleITK image.
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
Object SimpleITK image with identity direction.
- Return type:
SimpleITK Image
- fredtools.overwriteCTPhysicalProperties(img, RSfileName, areaFraction=0.5, CPUNo='auto', relElecDensCalib=[[-1024, -1000, -777.82, -495.34, -64.96, -34.39, -3.87, 51.92, 56.99, 226.05, 857.65, 1313, 8513, 12668, 25332], [0, 0, 0.19, 0.489, 0.949, 0.976, 1, 1.043, 1.053, 1.117, 1.456, 1.696, 3.76, 6.58, 9.09]], HUrange=[-2000, 50000], displayInfo=False)
Overwrite HU values in a CT image based on structures’ physical properties.
The function searches in a structure RS dicom file for structures with the physical property defined, maps each structure to the CT image defined as an instance of a SimpleITK 3D image, and replaces the Hounsfield Units (HU) values for voxels inside the structure. Only the relative electronic density physical property (‘REL_ELEC_DENSITY’) is implemented now, and it is converted to a HU value based on relative electronic density to HU calibration, given as relElecDensCalib parameter, whereas the missing values are interpolated linearly and rounded to the nearest integer HU value.
- Parameters:
img (SimpleITK 3D Image) – Object of a SimpleITK 3D image.
RSfileName (string) – Path String to dicom file with structures (RS file).
areaFraction (scalar, optional) – Fraction of pixel area occupancy to calculate binary mask. See mapStructToImg function for more information. (def. 0.5)
CPUNo ({'auto', 'none'}, scalar or None, optional) – Define if the multiprocessing should be used and how many cores should be exploited. See mapStructToImg function for more information. (def. ‘auto’)
relElecDensCalib (array_like, optional) – 2xN iterable (e.g. 2xN numpy array or list of two equal size lists) describing the calibration between HU values and relative electronic density. The first element (column) is describing the HU values and the second the relative electronic density. The missing values are interpolated linearly and if the user would like to use a different interpolation like spline or polynomial, it is advised to provide it explicitly for each HU value. The structures with the relative electronic density outside the calibration range will be skipped and a warning will be displayed. (def. [[-1024, -1000, -777.82, -495.34, -64.96, -34.39, -3.87, 51.92, 56.99, 226.05, 857.65, 1313, 8513, 12668, 25332] , [0, 0, 0.190, 0.489, 0.949, 0.976, 1, 1.043, 1.053, 1.117, 1.456, 1.696, 3.76, 6.58, 9.09]])
HUrange (2-element array_like, optional) – 2-element iterable of HU range to overwrite the physical properties. Only the structures that the HU values, derived from the calibration, are within the range (including the boundaries) will be overwritten. No warning will be displayed. (def. [-2000, 50000])
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
An object of a transformed SimpleITK 3D image.
- Return type:
SimpleITK 3D Image
See also
mapStructToImg
mapping a structure to an image to create a mask.
setValueMask
setting values of the image inside/outside a mask.
- fredtools.addMarginToMask(imgMask, marginLateral, marginProximal, marginDistal, lateralKernelType='circular', displayInfo=False)
Add lateral, proximal and distal margins to mask.
The function adds lateral, proximal and/or distal margins to a binary mask defined as an instance of a SimpleITK 3D image describing a binary mask. The lateral directions are defined in the X and Y axes, whereas the distal and proximal are along the Z axis. It is the user’s responsibility to transform the image into the correct view. Usually the ‘getImgBEV’ routine can be used to get the beam’s eye view.
- Parameters:
imgMask (SimpleITK 3D Image) – An object of a SimpleITK 3D image describing a binary mask.
marginLateral (scalar) – Lateral margin in the mask unit, usually in [mm]
marginProximal (scalar) – Proximal margin in the mask unit, usually in [mm]
marginDistal (scalar) – Distal margin in the mask unit, usually in [mm]
lateralKernelType ({'circular', 'box', 'cross'}, optional) – Kernel type for the lateral dilatation. (def. ‘circular’)
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
Object of a SimpleITK 3D image describing the diluted mask.
- Return type:
SimpleITK 3D Image
See also
mapStructToImg
mapping a structure to an image to create a mask.
getImgBEV
transform an image to Beam’s Eye View (BEV).
- fredtools.addGaussMarginToMask(imgMask, gaussSigma=6, fractionAtEdge=0.9, edgeDist=4, displayInfo=False)
Add Gaussian margin to mask.
The function adds a Gaussian margin to a binary mask defined as an instance of a SimpleITK image describing a binary mask. The Gaussian shape is defined by the sigma value, gaussSigma, and the distance from the mask, edgeDist, at which the Gaussian slope should reach the fraction fractionAtEdge. See the FREDtools web page for a more descriptive image.
- Parameters:
imgMask (SimpleITK Image) – An object of a SimpleITK 3D image describing a binary mask.
gaussSigma (scalar, optional) – Sigma of the Gaussian shape, usually in [mm]. (def. 6)
fractionAtEdge (scalar, optional) – Fraction of the Gaussian slope. (def. 0.9)
edgeDist (scalar, optional) – Distance from the mask edge, at which the Gaussian slope should reach the given fraction, usually in [mm]. (def. 4)
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
Object of a SimpleITK image describing the mask with a Gaussian margin.
- Return type:
SimpleITK Image
See also
addExpMarginToMask
add exponential margin to mask.
Notes
The distance from the binary mask is calculated with the SimpleITK.SignedDanielssonDistanceMap routine, which calculates the distance from the nearest mask voxel center and not from the voxel edge.
- fredtools.addExpMarginToMask(imgMask, exponent=0.25, edgeDist=4, displayInfo=False)
Add exponential margin to mask.
The function adds an exponential fall-off margin to a binary mask defined as an instance of a SimpleITK image describing a binary mask. The exponential fall-off shape is defined by the exponent parameter and the distance from the mask, edgeDist, at which the exponent starts. The exponent is described with the equation:
See the FREDtools web page for a more descriptive image.
- Parameters:
imgMask (SimpleITK Image) – An object of a SimpleITK image describing a binary mask.
exponent (scalar, optional) – Exponent value, usually in [1/mm]. (def. 0.25)
edgeDist (scalar, optional) – Distance from the mask edge, at which the exponential fall-off starts, usually in [mm]. (def. 4)
displayInfo (bool, optional) – Displays a summary of the function results. (def. False)
- Returns:
Object of a SimpleITK image describing the mask with an exponential margin.
- Return type:
SimpleITK Image
See also
addGaussMarginToMask
add Gaussian margin to mask.
Notes
The distance from the binary mask is calculated with the SimpleITK.SignedDanielssonDistanceMap routine, which calculates the distance from the nearest mask voxel center and not from the voxel edge.
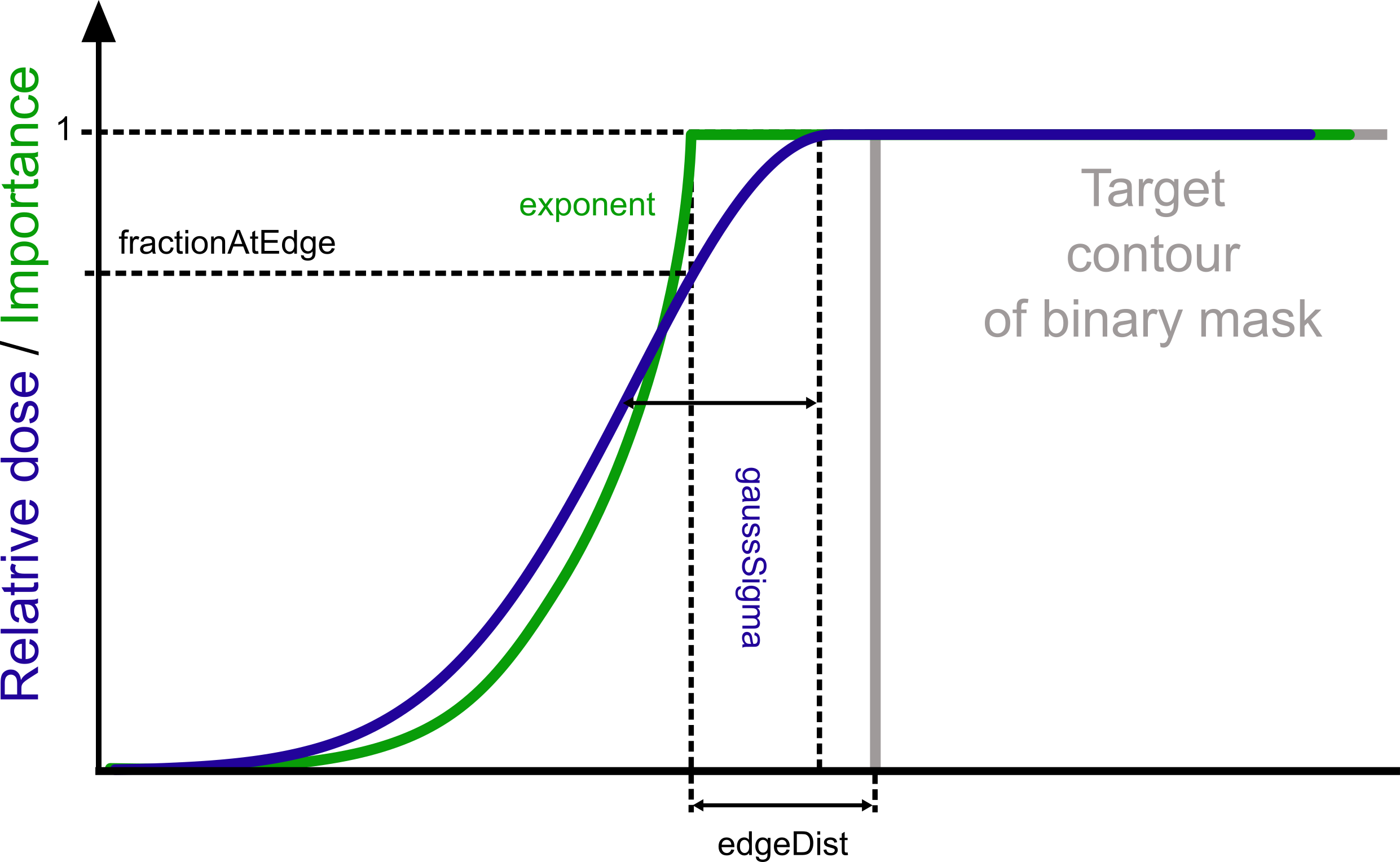
Definition of Gaussian and exponential margins.